C# Syllabus
Introduction
The W3Schools C# Tutorial is comprehensive and beginner-friendly.
It will give you a fundamental knowledge of C#.
It is designed for beginners and requires no prior experience with programming.
The content has been carefully made to be bite-sized, simple, and easy to understand.
The content has been proven by millions of users over the years. It is updated and improved frequently.
The syllabus outline and its sequence are structured so you can learn C# step by step, from the introduction to creating your first application with C#.
Learning Outcomes
- Understand C# and set up a development environment.
- Learn C#, including syntax, variables, data types, and operators.
- Use control structures such as if statements, for loops, and while loops.
- Understand and apply object-oriented programming (OOP) concepts: classes, objects, and inheritance.
- Create and use methods to organize your code and make it easier to understand.
- Handle errors in your code and write code to manage them properly.
- Write and run C# programs.
Note: Are you a teacher teaching C# programming? W3Schools Academy is a toolbox of features that can help you teach. It offers classroom features such as pre-built study plans, classroom administration and much more. Read more about Academy here.
Which Subjects Are C# Relevant For?
-
Computer Science:
C# is used to teach programming, software development, and object-oriented programming (OOP). -
Mathematics:
C# can be used for solving math problems and creating algorithms. -
Engineering:
C# is used for building software and tools for projects. -
Game Development:
C# is popular for creating games, particularly with game engines like Unity. -
Information Technology (IT):
C# is used to develop software applications and understand how systems work. -
Business and Data Analysis:
C# can be used to create applications for analyzing business data and for automating tasks. -
Web Development:
C# is used for building web applications and services using frameworks like ASP.NET. - C# can help you learn problem-solving and develop programming skills.
Activities
In this tutorial we offer different activities for you to learn C# for free:
Sign in to Track Progress
You can also create a free account to track your progress.
As a signed-in user, you get access to features such as:
- Learning paths
- Sandbox and lab environments
- Achievements
- And much more!
Overview of the Modules
- C# HOME
- C# Introduction
- C# Get Started
- C# Syntax
- C# Output
- C# Comments
- C# Variables
- C# Data Types
- C# Type Casting
- C# User Input
- C# Operators
- C# Math
- C# Strings
- C# Booleans
- C# If...Else (Conditions)
- C# Switch
- C# While Loop
- C# For Loop
- C# Break/Continue
- C# Arrays
- C# Methods
- C# Method Parameters
- C# Method Overloading
- C# OOP (Object-Oriented Programming)
- C# Classes/Objects
- C# Class Members
- C# Constructors
- C# Access Modifiers
- C# Properties
- C# Inheritance
- C# Polymorphism
- C# Abstraction
- C# Interface
- C# Enums
- C# Files
- C# Exceptions
Sandbox and Lab Environment
C#, like any other programming language, is best learned by working hands-on with code.
Easily try code with our "Try it Yourself" editor.
Here, you can edit C# code and view the result:
Example
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
}
}
}
If you want to explore more and host your project, we have a feature called Spaces that allows you to build, test and deploy C# code and other backend languages.
Here you get a secure sandbox environment called Spaces, where you can practice coding and test projects in real-time.
Spaces allow you to test, build, and deploy code. This includes a W3Schools subdomain, hosting, and secure SSL certificates.
Spaces require no installation and run directly in the browser.
Features include:
- Collaboration
- File navigator
- Terminal & log
- Package manager
- Database
- Environment manager
- Analytics
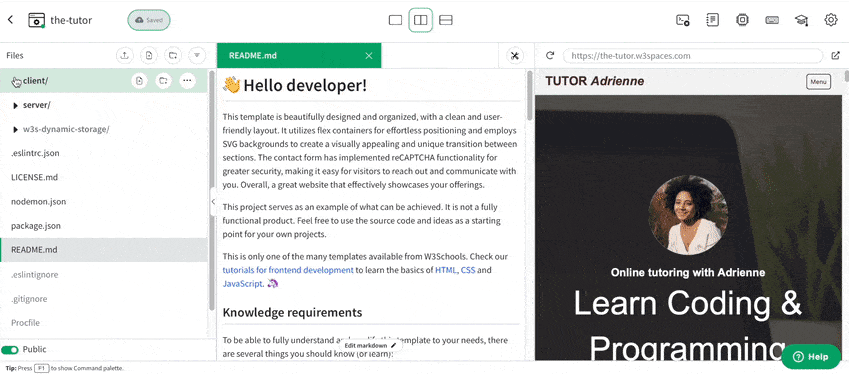
C# Certification
W3Schools offers an end-of-pathway certification program.
Here you can take exams to get certified.
The C# exam is a test that summarizes the W3Schools C# syllabus.
After passing the exam you get the "Certified C# Developer" Certification.
There are two different types of certifications:
- Non-adaptive
- Adaptive
The non-adaptive is pass or no pass.
The adaptive certification is adaptive and graded; students will get a grade from intermediate, advanced to professional.
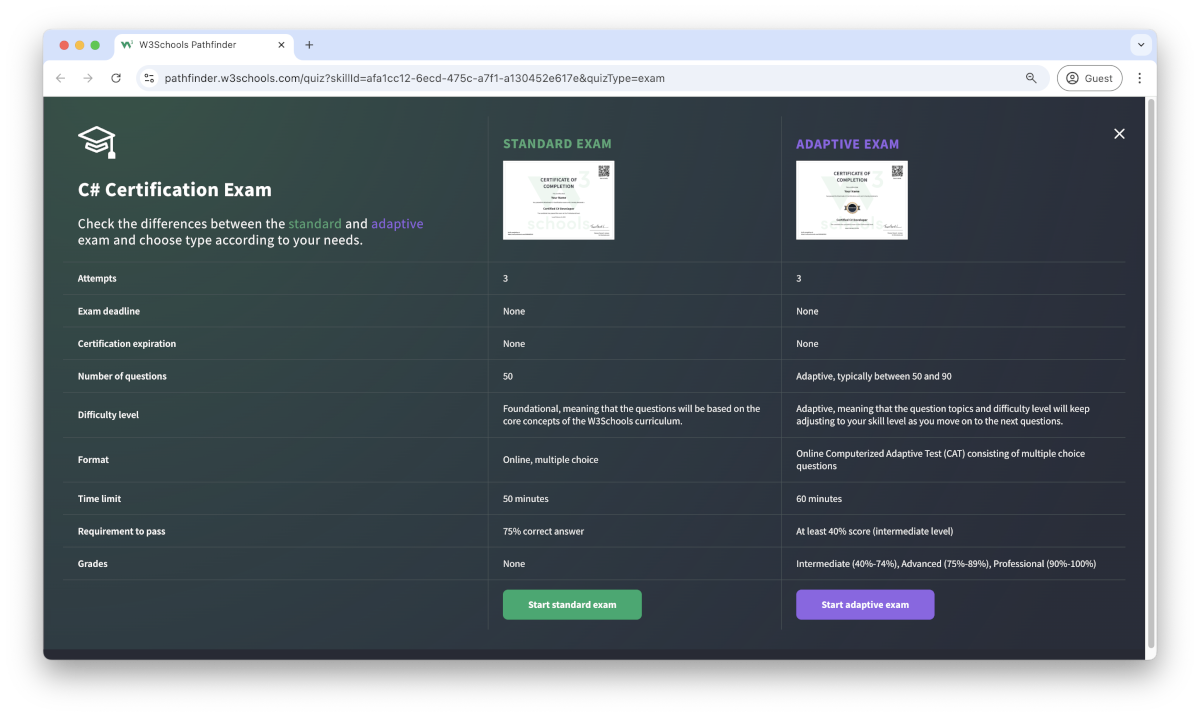
Are You a Teacher?
Are you interested in learning how you can use W3Schools Academy to Teach C#?
Watch a demo of W3Schools Academy. You'll see how it works, and discover how it can make teaching programming easier and more engaging.
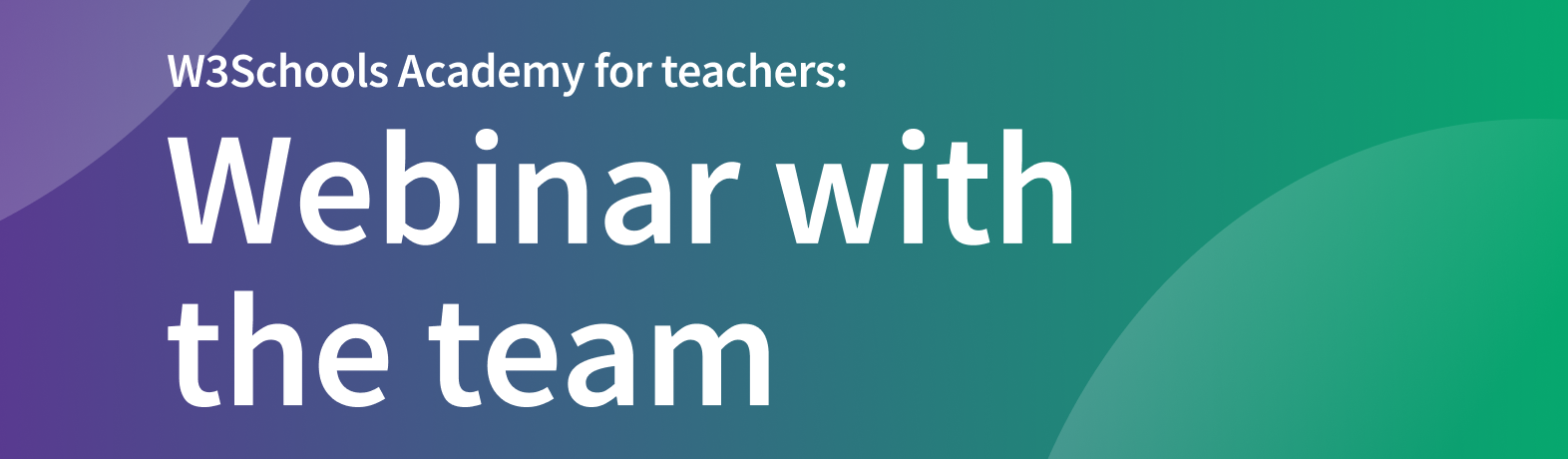