NumPy Syllabus
Introduction
The W3Schools NumPy Tutorial is comprehensive and beginner-friendly.
It will give you a fundamental knowledge of NumPy.
It is designed for beginners and requires only basic Python knowledge.
The content has been carefully made to be bite-sized, simple, and easy to understand.
The content has been proven by millions of users over the years. It is updated and improved frequently.
The syllabus outline and its sequence are structured so you can learn NumPy step by step, from the introduction to creating your first array operations.
Learning Outcomes
- Understand the basic structure of NumPy arrays.
- Create and manipulate arrays efficiently.
- Perform mathematical operations on arrays.
- Apply array indexing and slicing.
- Use array functions and methods.
- Perform array reshaping and stacking.
- Work with random number generation.
- Apply linear algebra operations.
- Create efficient numerical computations.
Note: Are you a teacher teaching NumPy? W3Schools Academy is a toolbox of features that can help you teach. It offers classroom features such as pre-built study plans, classroom administration and much more. Read more about Academy here.
Which Subjects Are NumPy Relevant For?
- Data Science:
NumPy is essential for numerical computing and data manipulation. - Scientific Computing:
NumPy provides powerful tools for scientific calculations and analysis. - Machine Learning:
NumPy is fundamental for handling numerical data in Machine Learning applications. - Statistics:
NumPy offers efficient tools for statistical calculations. - Image Processing:
NumPy arrays are perfect for handling image data. - Engineering:
NumPy supports mathematical operations for engineering applications. - Research:
NumPy is widely used in scientific research and data analysis.
Activities
In this tutorial we offer different activities for you to learn NumPy for free:
Sign in to Track Progress
You can also create a free account to track your progress.
As a signed-in user, you get access to features such as:
- Learning paths
- Sandbox and lab environments
- Achievements
- And much more!
Overview of the Modules
- NumPy HOME
- NumPy Intro
- NumPy Getting Started
- NumPy Creating Arrays
- NumPy Array Indexing
- NumPy Array Slicing
- NumPy Data Types
- NumPy Copy vs View
- NumPy Array Shape
- NumPy Array Reshape
- NumPy Array Iterating
- NumPy Array Join
- NumPy Array Split
- NumPy Array Search
- NumPy Array Sort
- NumPy Array Filter
- Random Intro
- Data Distribution
- Random Permutation
- Seaborn Module
- Normal Distribution
- Binomial Distribution
- Poisson Distribution
- Uniform Distribution
- Logistic Distribution
- Multinomial Distribution
- Exponential Distribution
- Chi Square Distribution
- Rayleigh Distribution
- Pareto Distribution
- Zipf Distribution
- ufunc Intro
- ufunc Create Function
- ufunc Simple Arithmetic
- ufunc Rounding Decimals
- ufunc Logs
- ufunc Summations
- ufunc Products
- ufunc Differences
- ufunc Finding LCM
- ufunc Finding GCD
- ufunc Trigonometric
- ufunc Hyperbolic
- ufunc Set Operations
Sandbox and Lab Environment
NumPy, like any other library, is best learned through hands-on practice.
Try this example using our editor:
ExampleGet your own Python Server
Create a NumPy array:
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
print(arr)
print(type(arr))
Try it Yourself »
If you want to explore more and host your project, we have a feature called Spaces that allows you to build, test and deploy Python projects for free.
Here you get a secure sandbox environment called Spaces, where you can practice NumPy code and test projects in real-time.
Spaces allow you to test, build, and deploy code. This includes a W3Schools subdomain, hosting, and secure SSL certificates.
Spaces require no installation and run directly in the browser.
Features include:
- Collaboration
- File navigator
- Terminal & log
- Package manager
- Database
- Environment manager
- Analytics
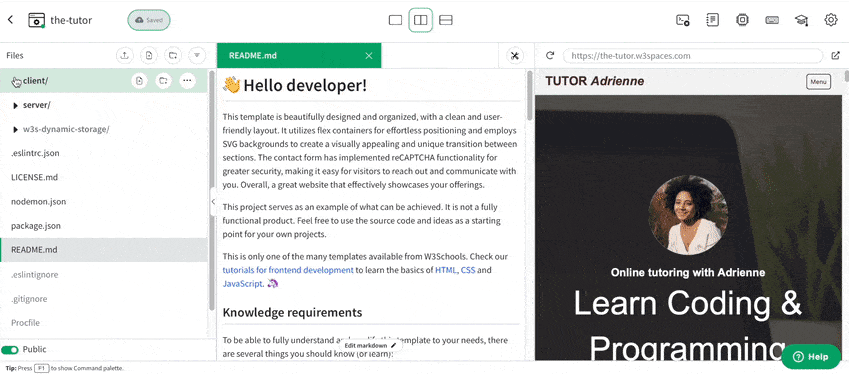
NumPy Certification
W3Schools offers an end-of-pathway certification program.
Here you can take exams to get certified.
The NumPy exam is a test that summarizes the W3Schools NumPy syllabus.
After passing the exam you get the "Certified NumPy Developer" Certification.
There are two different types of certifications:
- Non-adaptive
- Adaptive
The non-adaptive is pass or no pass.
The adaptive certification is adaptive and graded; students will get a grade from intermediate, advanced to professional.
Are You a Teacher?
Are you interested in learning how you can use W3Schools Academy to Teach NumPy?
Watch a demo of W3Schools Academy. You'll see how it works, and discover how it can make teaching programming easier and more engaging.
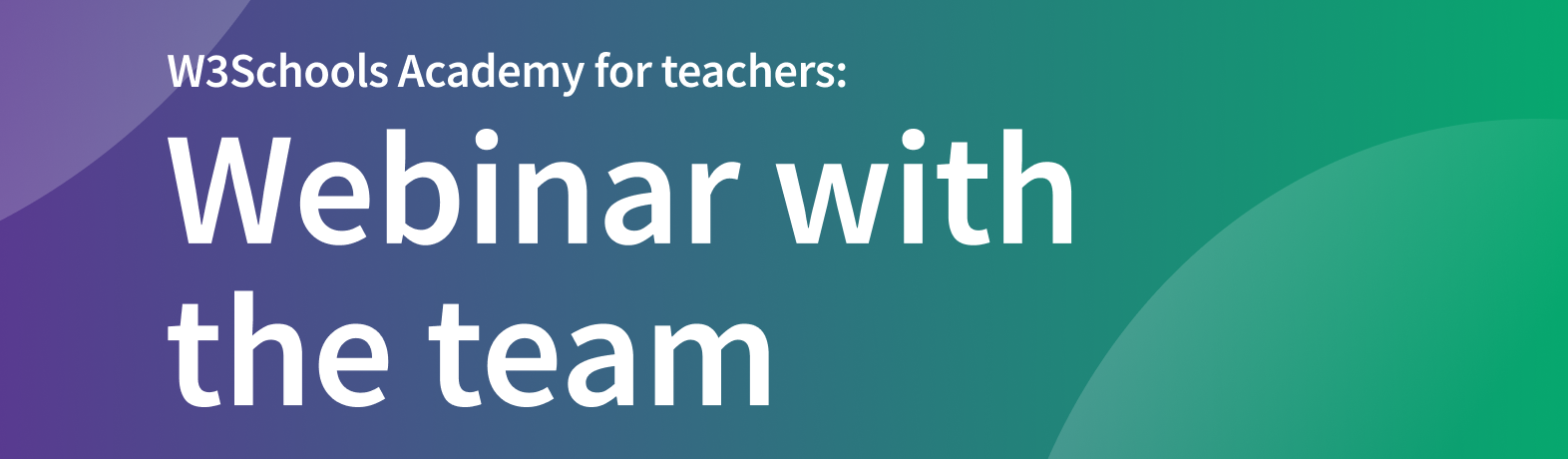